ADVENTURES IN GO: CLI FIBONACCI
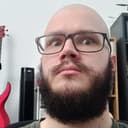
So my ‘first’ (It’s not really my first) adventure in Go I thought I’d attempt to make some sort of tool to generate a Fibonacci sequence. The idea is the if I call the program it will give me 10 numbers in the sequence or I can pass it a value and have it return that count of numbers.
It feels sort of overkill but I created a function to accept two ints, add them together, and then return the result.
Then I added some functionality to pass a number from the CLI as a flag or just default it to 10. then I just loop through until i reach that count.
In the loop i just output the current number we are at, add it to the last number we had (defaulting to 0 and 1). Set the last number to the current one and the current number to the value of the calculation so its ready for the next loop.
This seemed to work fine but feels far from ideal. I think it could be made a lot more efficient and also it can’t handle larger integers so this may be what I look at fixing next.
If you have any comments about how I approached this and any suggestions to improve it please leave a comment below! Your help on my adventure to learning Go is much appreciated.
package main
import (
"flag"
"fmt"
)
func main() {
count := flag.Int("n", 10, "fib count")
flag.Parse()
lastNumber := 0
currentNumber := 1
for index := 0; index < int(*count); index++ {
fmt.Println(currentNumber)
nextNumber := AddNumbers(lastNumber, currentNumber)
lastNumber = currentNumber
currentNumber = nextNumber
}
}
func AddNumbers(number1 int, number2 int) int {
return number1 + number2
}